[Flutter/dart]Create a text input form
- M.R
- Aug 15, 2021
- 2 min read
Overview
I will show you how to create a form for users to enter texts in the app.
Method
Create a new Stateful Widget. The reason is that I want to change whether the "OK" button can be pressed according to the entered content. (For example, on Twitter, you can't press the tweet button unless the number of characters is 1-140.)
class MyTextInput extends StatefulWidget{
final bool Function(String) buttonEnable;//Function to check the
//input string
String title; //Input form title
int maxLen; //Longest input string
MyTextInput({this.buttonEnable, this.title, this.maxLen});
@override
State<StatefulWidget> createState() {
return MyTextInputState();
}
}
class MyTextInputState extends State<MyTextInput>{
bool _enableButton=false;
TextEditingController controller;
@override
void initState() {
controller=TextEditingController();
}
@override
Widget build(BuildContext context) {
return AlertDialog(
title: Text(widget.title),
content: Column(
crossAxisAlignment: CrossAxisAlignment.center,
children:[
TextField(
controller: controller,
maxLength: widget.maxLen,
onChanged: (text){
_enableButton=widget.buttonEnable(text); //1
setState(() {});
},
),
SizedBox(height: 8,),
Row(
mainAxisAlignment: MainAxisAlignment.end,
children: [
FlatButton(
child: Text("Cancel"),
textColor: Colors.blue,
onPressed: ()async{Navigator.of(context).pop("");}
),
FlatButton(
child: Text("OK"),
textColor: Colors.blue,
onPressed: _enableButton? ()async{
Navigator.of(context).pop(controller.text);
}: null //2
),
],
),
]
)
);
}
}
This class takes three arguments. buttonEnable is a function that checks the input string. Check the input contents each time in the onChange event of TextField (1), and if there is a problem, set onPressed of FlatButton to null (2). By doing this, you will not be able to press the OK button if the input content is out of specification.
This class can be used as follows.
class MyHomePageState extends State<MyHomePage>{
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('demo'),
),
body: Container(
child: Center(
child: RaisedButton(
child: Text('input text'),
onPressed: ()async{
String input=await showInputTextForm(context);
//1 Process input
},
),
)
)
);
}
Future<String> showInputTextForm(BuildContext context)async{
String input=await showDialog<String>(
context: context,
builder:(context)=> MyTextInput(buttonEnable: checkInput, maxLen: 100, title: "入力",)
);
if (input==null){
input="";
}
return input;
}
bool checkInput(String text){
return text.length>0;
}
}
In this example, if the number of characters is 0 or more, you can press the OK button. Of course, more complicated processing is possible (such as the upper limit of the number of characters and whether there is any duplication with existing data).
Write the process according to the input character string obtained in part 1. When the Cancel button is pressed, a blank character string will be returned, so please separate the cases.
An input form like this will be displayed. (The OK button is disabled because I haven't entered anything)
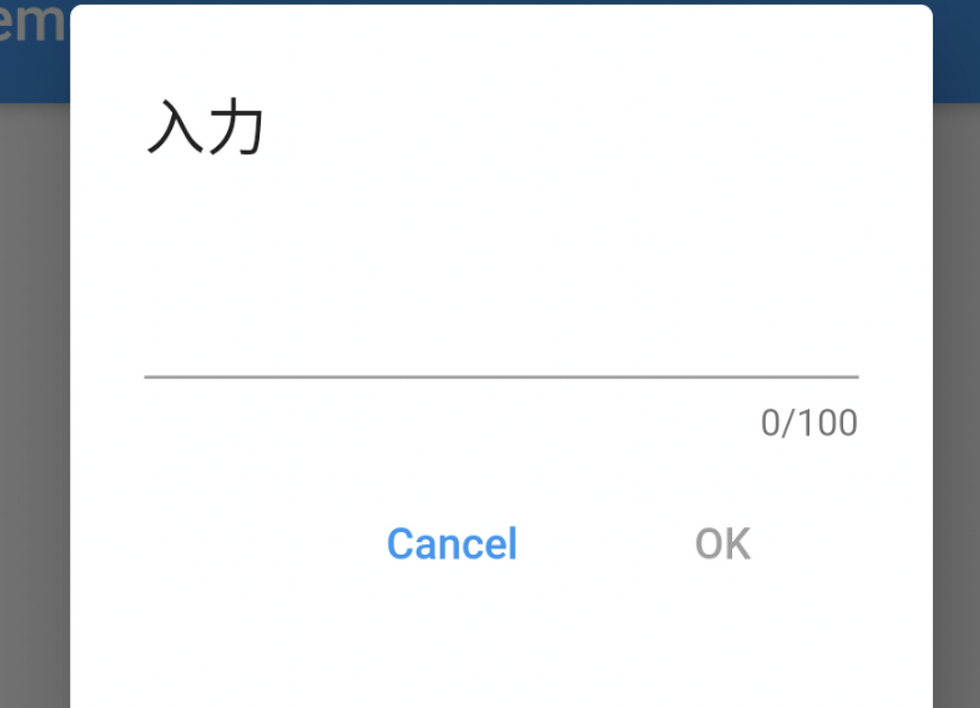
Recent Posts
See AllWhat want to do I want to create an input form using TextField. For example, if the input content is a monetary amount, I would like to...
What want to do There is a variable that remain unchanged once the initial value is determined. However, it cannot be determined yet when...
What want to do As the title suggests. Place two widgets in one line on the screen One in the center of the screen and the other on the...
Commentaires