[Flutter/Dart] Numbering of id when adding a new item
- M.R
- Jul 17, 2023
- 2 min read
Issue
Assumptions
Register and save multiple items
Items are managed with a unique id
State management is redux The flow of adding a new item is as follows
Dispatch AddItemAction with the input from the user as an argument in the view
Register {id, item} in store with reducer
Register {id, item} in remote database with middleware

Issue
Where to number the id when adding a new itemAccording to the flow above, there are three candidates for numbering: view, reducer, and middleware.
Solution
I think it's better to use middleware for numbering.
When numbering in view, there are the following problems.
Duplicate processing when adding items from multiple different pages or UIs
Id is a variable related to state management within the app, and the view should not know it (separation of concerns)
When numbering with reducer, there are the following problems.
Middleware can't know new id
Details
In the following, I will describe the detail method to add with middleware.
The biggest issue is how to convey the id numbered by middleware to the reducer.
To deal with this:
Define AddItemAction(Item) and AddItemWithIdAction(Item, int)
View dispatch AddItemAction based on user input
When middleware detects AddItemAction, it numbers a new id and dispatches AddItemWithIdAction
Reducer does nothing for AddItemAction. When AddItemWithIdAction is detected, add argument id and item to store
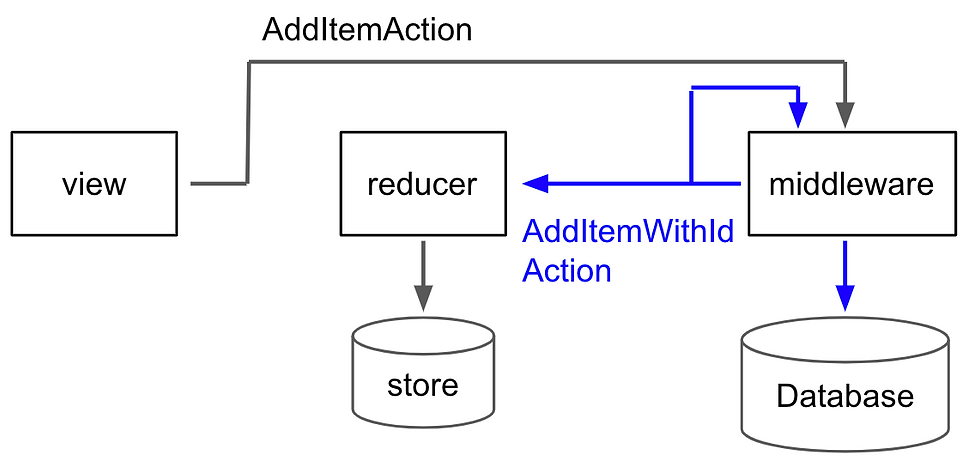
The code looks like this:
//Action
class AddItemAction{
final Item item;
AddItemAction(this.item);
}
class AddItemWithIdAction{
final Item item;
final int id;
AddItemWithIdAction({required this.id, required this.item});
}
//middleware
void AppStateMiddleWare(Store<AppState> store, dynamic action,
NextDispatcher next) async {
if(action is AddItemAction){
int id = getId(); //number new id
store.dispatch(
AddItemWithIdAction(id: id, item: action.item)
);
}
next(action);
if (action is AddItemWithIdAction){
saveToDB(action.id, action.item); //save to database
}
}
AppState appStateReducer(AppState state, action){
if (action is AddItemWithIdAction){
return addItem(action.id, action.item, state);
//save {id, item} to store
}
return state;
}
Lastly
If you try to follow the framework, there will be various restrictions.
Recent Posts
See AllWhat want to do I want to create an input form using TextField. For example, if the input content is a monetary amount, I would like to...
What want to do There is a variable that remain unchanged once the initial value is determined. However, it cannot be determined yet when...
What want to do As the title suggests. Place two widgets in one line on the screen One in the center of the screen and the other on the...
Comments