[Flutter/dart]Sorting and extracting Map elements
- M.R
- Aug 13, 2021
- 2 min read
Overview
When creating an application with Flutter, I often store user data etc. in Map (because it is troublesome to deal with index deviation when deleting data with list).
In that case, when displaying the data on the screen, it is necessary to devise to specify the order or extract only the data under specific conditions. (C # dictionary can be treated like list ...)
Method
If you convert Map to List of MapEntry, you can use methods such as where() and sort(). After that, the argument should be taken in List <MapEntry> on the user side (basically it should be build() of Widget).
sorting
In the following, the comparison method is passed as a delegate so that it can handle multiple sorting methods.
class UserData{
String name;
int score;
UserData({this.name, this.score});
}
class MyHomePageState extends State<MyHomePage> {
Map<int, UserData> data;
@override
void initState() {
data={
0: UserData(name: "Alice", score: 90),
1: UserData(name: "Bob", score: 87),
2: UserData(name: "Charlie", score: 98),
};
}
@override
Widget build(BuildContext context) {
//Sort in ascending order of score
var _entries=_orderData(data, (a, b) => (a.value as
UserData).score.compareTo((b.value as UserData).score));
return Scaffold(
appBar: AppBar(
title: Text('demo'),
),
body: Container(
padding: const EdgeInsets.all(16),
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: _entries.map((e) => ListTile(
title: Text(
"${e.value.name}: ${e.value.score}",
style: TextStyle(
fontSize: 16
),
),
)).toList(),
)
)
);
}
//this!
List<MapEntry> _orderData(Map<int, dynamic>maps, int
Function(MapEntry<int, dynamic>, MapEntry<int, dynamic>) sorter){
return maps.entries.toList()..sort((a, b)=> sorter(a, b));
}
}
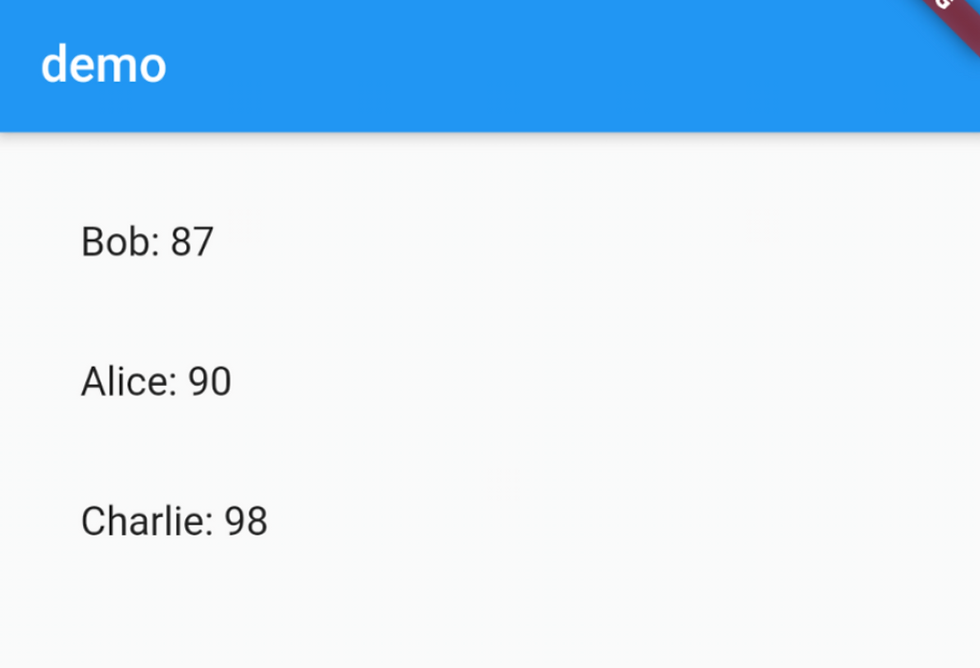
extract
Similarly, create a method that takes a delegate that represents the condition as an argument.
List<MapEntry> _selectData(Map maps, bool Function(MapEntry) predicate){
return maps.entries.toList().where((element) =>
predicate(element)).toList();
}
@override
Widget build(BuildContext context) {
//Extract data with a score of 90 or higher
var _entries=_selectData(data, (e) => e.value.score>90);
return Scaffold(
appBar: AppBar(
title: Text('demo'),
),
body: Container(
padding: const EdgeInsets.all(16),
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: _entries.map((e) => ListTile(
title: Text(
"${e.value.name}: ${e.value.score}",
style: TextStyle(
fontSize: 16
),
),
)).toList(),
)
)
);
}
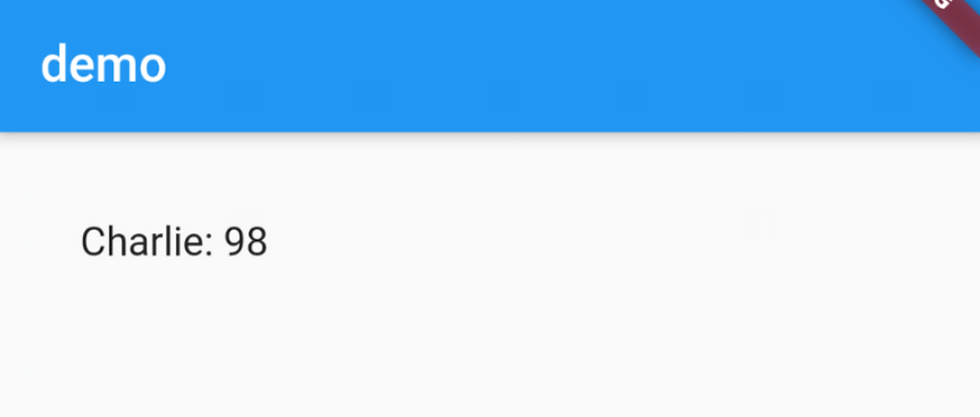
Lastly
I use Map for ease of searching and deleting, and List <MapEntry> for easy sorting and condition extraction when listing in widget .
Recent Posts
See AllWhat want to do I want to create an input form using TextField. For example, if the input content is a monetary amount, I would like to...
What want to do There is a variable that remain unchanged once the initial value is determined. However, it cannot be determined yet when...
What want to do As the title suggests. Place two widgets in one line on the screen One in the center of the screen and the other on the...
Comments