[Flutter/dart] Table is useful to align Widget
- M.R
- Aug 8, 2021
- 2 min read
Overview
When aligning the positions of multiple widgets with Flutter, I think that you often use Column for vertical alignment and Row for horizontal alignment. However, when you want to align both the vertical and horizontal positions, it may be difficult to use both Row and Column together. In such a case, it is convenient to use Table.
Example
For example, suppose you want Widgets A and B to be placed horizontally and centered vertically, and Widget C to be centered horizontally below Widget B.
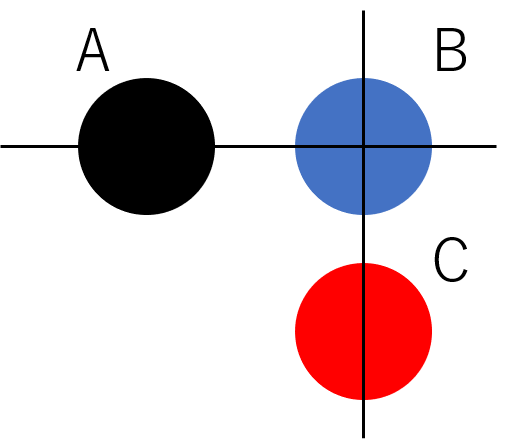
At this time, if A and B are put in Row, B and C cannot be centered horizontally. It is possible to make adjustments with SizedBox, but it will probably shift when the screen size changes.
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('demo'),
),
body: Container(
padding: const EdgeInsets.all(16),
child: Column(
children: [
Row(
children: [
widgetA(),
SizedBox(width: 16,),
widgetB()
],
),
SizedBox(height: 16,),
Row(
children: [
SizedBox(width: 40,),
widgetC()
],
)
],
)
)
);
}
Widget widgetA(){
return Icon(
Icons.calendar_today,
color: Colors.black,
);
}
Widget widgetB(){
return Icon(
Icons.help,
color: Colors.blue,
);
}
Widget widgetC(){
return Icon(
Icons.edit,
color: Colors.red,
);
}
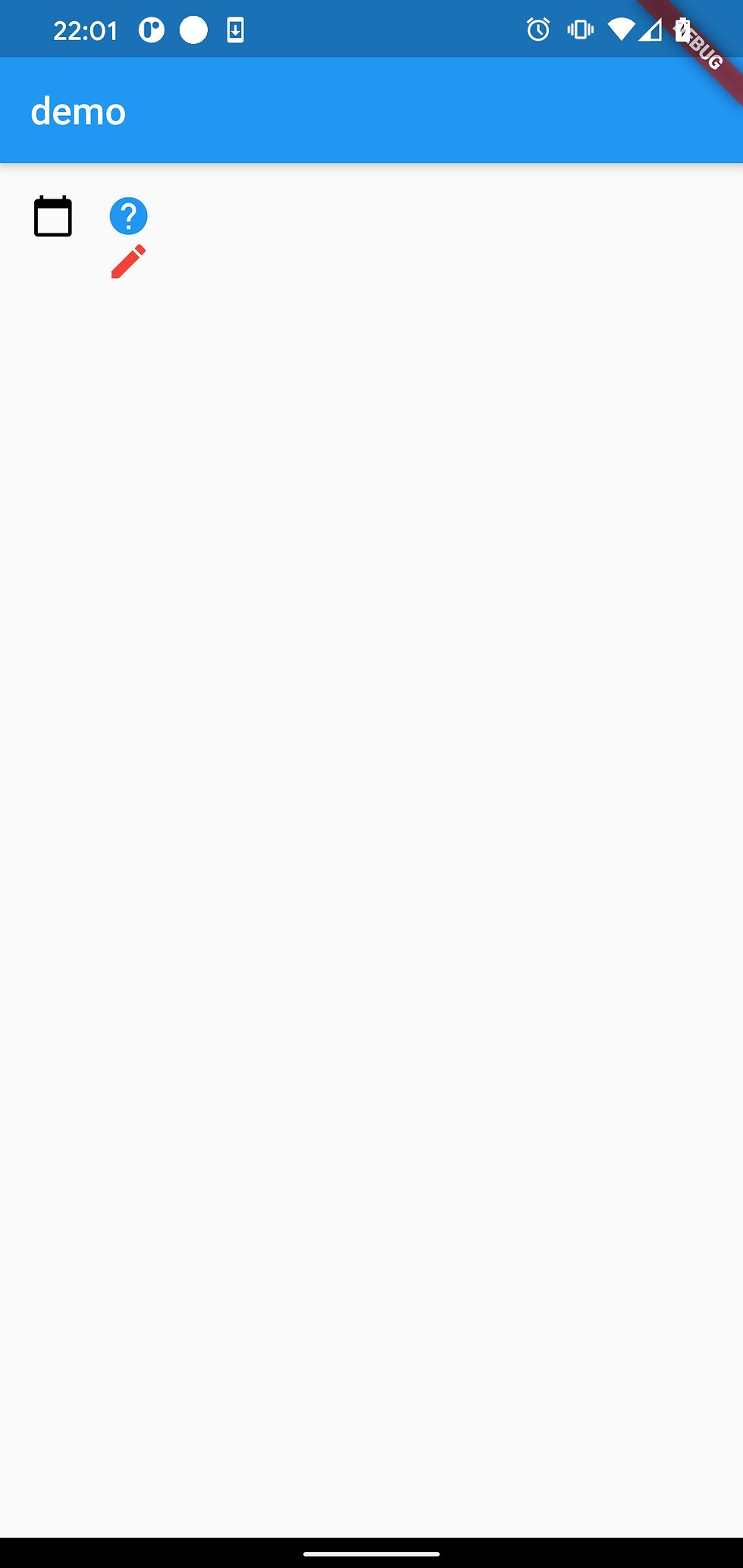
Also, if B and C are put in Column, A and B cannot be centered vertically.
In this case, use Table.
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('demo'),
),
body: Container(
padding: const EdgeInsets.all(16),
child: Table(
defaultColumnWidth: IntrinsicColumnWidth(),
children: [
TableRow(
children: [
widgetA(),
SizedBox(width: 16,),
widgetB()
],
),
TableRow(
children: [
SizedBox(width: 0,),
SizedBox(width: 16,),
widgetC()
]
)
],
)
)
);
}
Put the widgets you want to line up horizontally in TableRow.
This time, I want widgetC to be under widgetB, so I will adjust it by putting a SizedBox with a width of 0 in the part under widgetA.
The width of each column is determined by the defaultColumnWidth. This time it fits the width of the child widget (IntrinsicColumnWidth ()).
With this, you can easily adjust the position!

Summary
Consider Table if you want to align your widgets both vertically and horizontally!
Recent Posts
See AllWhat want to do I want to create an input form using TextField. For example, if the input content is a monetary amount, I would like to...
What want to do There is a variable that remain unchanged once the initial value is determined. However, it cannot be determined yet when...
What want to do As the title suggests. Place two widgets in one line on the screen One in the center of the screen and the other on the...
Comments